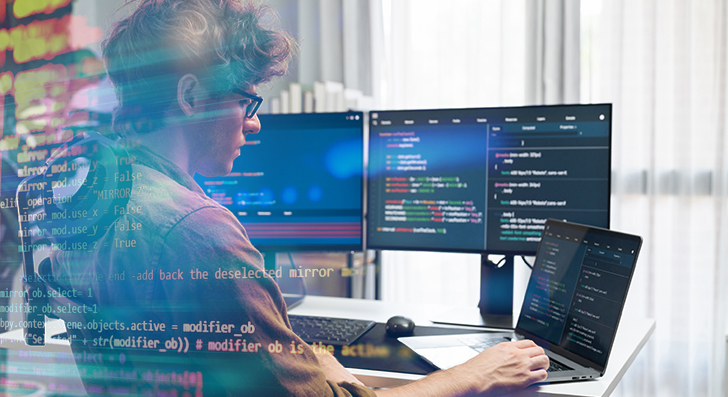
Scalability signifies your software can cope with progress—a lot more customers, extra facts, and a lot more site visitors—with out breaking. As a developer, making with scalability in mind will save time and anxiety later on. Here’s a transparent and sensible guideline that may help you commence by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability isn't some thing you bolt on afterwards—it should be aspect of one's system from the beginning. Quite a few programs are unsuccessful once they grow rapid simply because the initial design can’t take care of the extra load. Like a developer, you might want to Feel early about how your technique will behave stressed.
Begin by coming up with your architecture to become versatile. Stay clear of monolithic codebases in which anything is tightly connected. In its place, use modular style or microservices. These designs crack your application into smaller, impartial sections. Each module or support can scale By itself with out impacting The full procedure.
Also, consider your database from day just one. Will it need to handle a million buyers or just a hundred? Choose the appropriate form—relational or NoSQL—dependant on how your data will increase. Program for sharding, indexing, and backups early, even if you don’t need to have them still.
Another essential level is in order to avoid hardcoding assumptions. Don’t produce code that only is effective less than current ailments. Think about what would happen In case your user base doubled tomorrow. Would your app crash? Would the database slow down?
Use style patterns that aid scaling, like message queues or event-driven units. These help your app manage a lot more requests with no receiving overloaded.
When you build with scalability in mind, you are not just planning for fulfillment—you happen to be cutting down foreseeable future complications. A effectively-prepared process is simpler to keep up, adapt, and develop. It’s better to prepare early than to rebuild afterwards.
Use the appropriate Databases
Selecting the right database is usually a critical Portion of developing scalable purposes. Not all databases are created precisely the same, and using the Completely wrong you can sluggish you down or even induce failures as your application grows.
Start by being familiar with your knowledge. Is it really structured, like rows in the table? If Of course, a relational database like PostgreSQL or MySQL is a good healthy. These are generally strong with interactions, transactions, and consistency. Additionally they support scaling approaches like go through replicas, indexing, and partitioning to take care of extra targeted traffic and data.
If the information is much more adaptable—like consumer exercise logs, item catalogs, or files—consider a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing big volumes of unstructured or semi-structured knowledge and will scale horizontally a lot more quickly.
Also, think about your read through and write patterns. Will you be carrying out numerous reads with fewer writes? Use caching and read replicas. Do you think you're managing a hefty publish load? Take a look at databases that may manage significant compose throughput, or maybe party-primarily based info storage devices like Apache Kafka (for non permanent data streams).
It’s also intelligent to Feel forward. You might not will need Superior scaling characteristics now, but picking a databases that supports them usually means you received’t need to switch later.
Use indexing to speed up queries. Steer clear of needless joins. Normalize or denormalize your facts based upon your obtain styles. And always keep track of database overall performance as you develop.
In brief, the proper database is dependent upon your app’s construction, speed requirements, and how you expect it to grow. Take time to select correctly—it’ll help save a great deal of problems later on.
Enhance Code and Queries
Quickly code is key to scalability. As your application grows, each individual smaller delay adds up. Improperly penned code or unoptimized queries can decelerate functionality and overload your process. That’s why it’s crucial that you Construct effective logic from the beginning.
Start out by composing thoroughly clean, simple code. Stay clear of repeating logic and remove something unnecessary. Don’t pick the most advanced Resolution if a simple a person performs. Keep your capabilities limited, focused, and straightforward to test. Use profiling applications to seek out bottlenecks—locations exactly where your code usually takes way too lengthy to operate or makes use of too much memory.
Following, take a look at your databases queries. These frequently gradual issues down much more than the code alone. Make certain Each individual query only asks for the information you actually need to have. Avoid Decide on *, which fetches everything, and alternatively select distinct fields. Use indexes to hurry up lookups. And prevent doing too many joins, Primarily across massive tables.
For those who recognize the exact same data currently being asked for again and again, use caching. Retailer the final results temporarily working with applications like Redis or Memcached which means you don’t really need to repeat highly-priced operations.
Also, batch your database operations if you can. In lieu of updating a row one by one, update them in groups. This cuts down on overhead and helps make your application more effective.
Remember to take a look at with significant datasets. Code and queries that work good with one hundred data could crash every time they have to take care of one million.
In short, scalable apps are rapidly applications. Maintain your code restricted, your queries lean, and use caching when wanted. These ways assistance your software continue to be smooth and responsive, even as the load increases.
Leverage Load Balancing and Caching
As your app grows, it's got to handle a lot more end users and a lot more targeted traffic. If anything goes by just one server, it can promptly turn into a bottleneck. That’s the place load balancing and caching are available in. These two resources assist keep your app fast, secure, and scalable.
Load balancing spreads incoming targeted visitors throughout a number of servers. As an alternative to a single server carrying out all of the function, the load balancer routes users to distinctive servers based upon availability. What this means is no single server gets overloaded. If one server goes down, the load balancer can mail visitors to the Other individuals. Resources like Nginx, HAProxy, or cloud-centered alternatives from AWS and Google Cloud make this simple to setup.
Caching is about storing details briefly so it may be reused quickly. When people request the same info all over again—like a product page or maybe a profile—you don’t must fetch it from the databases each time. You could serve it from the cache.
There are 2 typical sorts of caching:
1. Server-facet caching (like Redis or Memcached) outlets information in memory for speedy accessibility.
two. Consumer-facet caching (like browser caching or CDN caching) shops static data files close to the consumer.
Caching reduces database load, increases speed, and would make your app extra productive.
Use caching for things which don’t alter generally. And usually ensure that your cache is updated when knowledge does change.
In brief, load balancing and caching are very simple but potent instruments. Together, they help your application tackle far more users, remain rapid, and Get better from difficulties. If you intend to mature, you'll need equally.
Use Cloud and Container Applications
To build scalable programs, you may need applications that let your app expand simply. That’s where cloud platforms and containers are available in. They provide you overall flexibility, lower set up time, and make scaling much smoother.
Cloud platforms like Amazon Internet Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and expert services as you need them. You don’t have to purchase hardware or guess potential capability. When targeted visitors improves, you can add more resources with just a few clicks or immediately utilizing auto-scaling. When visitors drops, you are able to scale down to save money.
These platforms also offer services like managed databases, storage, load balancing, and security tools. You could deal with developing your app instead of running infrastructure.
Containers are A further critical Device. A container packages your app and all the things it ought to operate—code, libraries, settings—into one device. This causes it to be straightforward to move your application amongst environments, out of your laptop computer to the cloud, without the need of surprises. Docker is the preferred Device for this.
When your application makes use of numerous containers, applications like Kubernetes make it easier to control them. Kubernetes handles get more info deployment, scaling, and recovery. If just one portion of your application crashes, it restarts it instantly.
Containers also make it very easy to independent elements of your application into providers. You can update or scale sections independently, which can be perfect for efficiency and reliability.
In a nutshell, using cloud and container instruments indicates you may scale quick, deploy conveniently, and Recuperate promptly when difficulties materialize. If you'd like your application to increase without limitations, get started making use of these instruments early. They save time, lessen hazard, and enable you to continue to be focused on creating, not correcting.
Monitor Every little thing
For those who don’t keep track of your application, you gained’t know when matters go Incorrect. Monitoring helps you see how your app is undertaking, location problems early, and make greater conclusions as your application grows. It’s a key Portion of constructing scalable units.
Start by tracking simple metrics like CPU utilization, memory, disk Place, and reaction time. These show you how your servers and services are performing. Equipment like Prometheus, Grafana, Datadog, or New Relic can assist you accumulate and visualize this data.
Don’t just keep track of your servers—check your app way too. Control just how long it will require for people to load internet pages, how frequently glitches materialize, and where by they manifest. Logging applications like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s going on within your code.
Arrange alerts for vital complications. Such as, In the event your reaction time goes earlier mentioned a Restrict or simply a company goes down, it is best to get notified promptly. This can help you correct concerns quickly, frequently prior to users even see.
Checking is additionally helpful whenever you make changes. For those who deploy a different characteristic and see a spike in faults or slowdowns, you may roll it back again before it results in true problems.
As your app grows, traffic and facts boost. With out checking, you’ll skip indications of difficulties till it’s much too late. But with the best resources set up, you remain on top of things.
In a nutshell, checking will help you keep your application reliable and scalable. It’s not almost spotting failures—it’s about knowledge your method and ensuring that it works very well, even under pressure.
Remaining Ideas
Scalability isn’t only for large providers. Even little applications require a robust Basis. By developing diligently, optimizing properly, and utilizing the right equipment, you can Construct applications that develop efficiently without the need of breaking under pressure. Start off small, Feel major, and build sensible.